Static Image Trajectory Rendering Example¶
Copyright (c) 2014-2020 National Technology and Engineering Solutions of Sandia, LLC. Under the terms of Contract DE-NA0003525 with National Technology and Engineering Solutions of Sandia, LLC, the U.S. Government retains certain rights in this software.
Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met:
1. Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer.
2. Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS “AS IS” AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
Imports
[1]:
from tracktable.domain import terrestrial
from tracktable.render import mapmaker
from tracktable.core import data_directory
from tracktable.render import paths
import matplotlib
from matplotlib import pyplot
import cartopy
import cartopy.crs
import os.path
Requirements: We will need data points built into trajectories. Replace the following with your own code to build the trajectories or use the provided example.
[2]:
trajectory_filename = os.path.join(data_directory(), 'SampleTrajectories.traj')
infile = open(trajectory_filename, 'r')
trajectories = terrestrial.TrajectoryReader()
trajectories.input = infile
[3]:
# JUPYTER NOTE: Jupyter will show you the state of the figure when you exit
# the cell in which you created it. You cannot apply different effects in
# different cells as far as I know. To work around this, just put all your
# different things in functions, then call those functions one after another
# in a single cell.
# Set up the canvas and map projection
# 8 x 6 inches at 100 dpi = 800x600 image
figure = pyplot.figure(dpi=100, figsize=(8, 6))
(mymap, map_actors) = mapmaker.mapmaker(domain='terrestrial',
map_name='region:world')
paths.draw_traffic(traffic_map = mymap, trajectory_iterable = trajectories, transform=cartopy.crs.PlateCarree())
[3]:
[<matplotlib.collections.LineCollection at 0x7f79df707550>,
<matplotlib.collections.PathCollection at 0x7f79df707d50>]
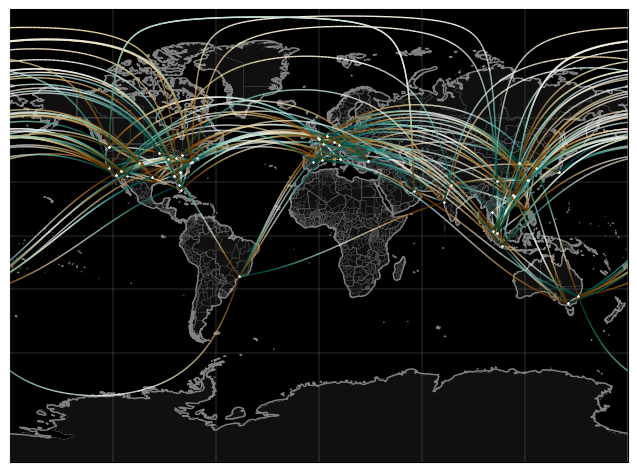